How to use the 2Captcha solver extension in Puppeteer for bypass reCAPTCHA and others captchas
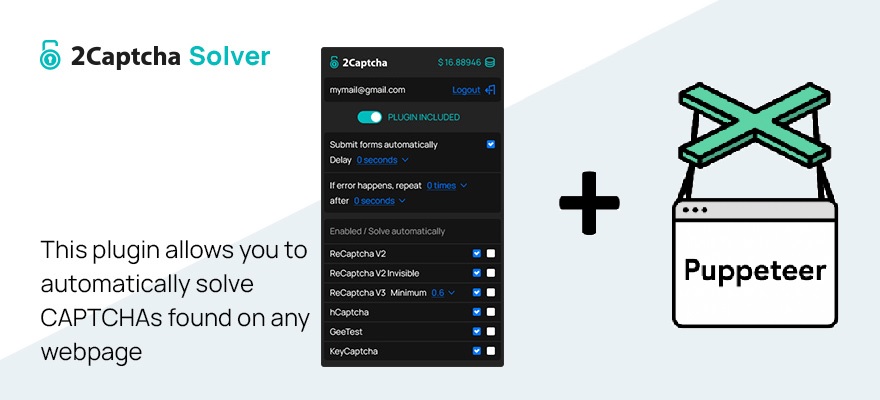
Using the extension and Puppeteer you can create a Puppeteer captcha solver solution.
In this article we will describe how to use the 2captcha-solver extension together with Puppeteer for bypass reCAPTCHA and any other typs of captchas. Puppeteer is a Node library that allows you to launch a browser and perform various actions in it, usage examples are described here. Puppeteer Node.js library is a great tool for a variety of tasks that require browser automation. Advantage of Puppeteer is its simplicity and the ability to work in headless
mode. There are plugins for Puppeteer that allow you to hide the fact of automation. Hiding the fact of automation is important when development parsers, as this will allow the browser to look more like a person and not be exposed.
In this article we will use Puppeteer, puppeteer-extra and puppeteer-extra-plugin-stealth. puppeteer-extra is a lightweight wrapper around Puppeteer, and puppeteer-extra-plugin-stealth is an addition to puppeteer-extra designed to hide traces of automation.
In the step-by-step description below, we will describe how to solve the captcha in on the page https://2captcha.com/demo/recaptcha-v2.
1. Installing components
Installing Puppeteer and other required packages:
npm i puppeteer puppeteer-extra puppeteer-extra-plugin-stealth
2. Setting up the extension
Download archive with the extension, and unzip it to the folder ./2captcha-solver
in the root of the project.
The extension has many settings, including automatic solution of the specified type of captcha, support for proxy
, and other settings. The settings are available in the file ./common/config.js
. To add settings for the automatic reCAPTCHA V2 solution, you need to open the file ./common/config.js
and change the value of the autoSolveRecaptchaV2
field to true
.
Next, you need to configure the extension:
-
Enter your API key in the extension settings file
./common/config.js
. Your key must be written to the value of theapiKey
field. You can see and copy you're API key on the page.Example:
apiKey: "8080629c1221fdd82m8080000ff0c99c"
-
Disable opening the extension settings page after installation. To do this, in the file
./manifest.json
delete the following lines:
"options_ui": {
"page": "options/options.html",
"open_in_tab": true
},
3. Browser Automation
Launching and initializing the extension in Puppeteer:
const puppeteer = require('puppeteer-extra');
const StealthPlugin = require('puppeteer-extra-plugin-stealth');
const { executablePath } = require('puppeteer');
(async () => {
const pathToExtension = require('path').join(__dirname, '2captcha-solver');
puppeteer.use(StealthPlugin())
const browser = await puppeteer.launch({
headless: false,
args: [
`--disable-extensions-except=${pathToExtension}`,
`--load-extension=${pathToExtension}`,
],
executablePath: executablePath()
});
const [page] = await browser.pages()
})();
3.1 Opening a page
Opening a page https://2captcha.com/demo/recaptcha-v2, and sending a captcha.
Using page.goto()
we go to the page https://2captcha.com/demo/recaptcha-v2. Next, you need to send a captcha for a solution, this can be done manually or automatically.
In our example, we will send a captcha manually, for this we wait until the extension button with the CSS selector .captcha-solver
is available, then click on this button. After clicking on the button, the captcha will go to the service for a solution.
// Opening a page
await page.goto('https://2captcha.com/demo/recaptcha-v2')
// Waiting for the element with the CSS selector ".captcha-solver" to be available
await page.waitForSelector('.captcha-solver')
// Click on the element with the specified selector
await page.click('.captcha-solver')
3.2 Checking the captcha status
After receiving a response from the service, the extension button .captcha-solver
will change the value of the data attribute data-state
. By observing the value of this data-state
attribute, you can monitor the state of the extension. After solving the captcha, the value of this attribute will change to "solved"
.
Description of the values of the data-state
attribute:
Attribute | Description |
---|---|
data-state="ready" |
The extension is ready to solve the captcha. To send a captcha, you need to click on the button. |
data-state="solving" |
Solving the captcha. |
data-state="solved" |
Captcha has been successfully solved |
data-state="error" |
An error when receiving a response or a captcha was not successfully solved. |
At this step, you need to wait until the captcha is solved, after that the attribute value will change to "solved"
, this will signal the successful solution of the captcha. After this step, you can do the necessary actions.
// By default, waitForSelector waits for 30 seconds, but this time is usually not enough, so we specify the timeout value manually with the second parameter. The timeout value is specified in "ms".
await page.waitForSelector(`.captcha-solver[data-state="solved"]`, {timeout: 180000})
4. Performing actions
After solving the captcha, we can start doing the necessary actions on the page. In our example, we will click on the "Check" button to check the correctness of the received captcha solution. After successfully passing the check, you will see the message "Captcha is passed successfully!".
// Click on the "Check" button to check the successful solution of the captcha.
await page.click("button[type='submit']")
Congratulations, the captcha has been successfully passed! The full source code of the example is here.
Video: How to solver captcha in Puppeteer
The video below shows how to use the extension in puppeteer.
Useful information:
-
The extension has many settings, for example, automatic solution of the specified type of captcha or support for
proxy
, all these settings are available in the file./common/config.js
, in the folder with the unpacked extension. To automatically send reCAPTCHA V2, in the file./common/config.js
change the value of theautoSolveRecaptchaV2
field totrue
. -
You can use sandbox mode, for testing. In sandbox mode, sent captchas will be sent to you. Learn more about sandbox you can read here.
-
Puppeteer running in
headless
mode does not support loading extension, to solve this problem you can use xvfb.
Useful links:
- The full source code of the example is here.
- 2captcha-solver (source code)
- 2captcha-solver (chrome web store)
- FAQ about the 2captcha service for developers https://2captcha.com/support/faq/developer
- puppeteer
- puppeteer-extra
- puppeteer-extra-plugin-stealth
- xvfb